1. Lecture, Labs, and Homeworks
Lectures, labs, and homeworks are all inter-related to deliver the course material. Labs will extend lecture concepts with hands of algorithmic practice and programming.
Most of your lab time will be spent working on problems in groups. However, it is still important that you pay attention to what is said by the instructor, as all material presented is important.
Finally, participation in lab is worth a portion of your grade in the course. You need to be in lab and working on the lab activities to get a good participation grade.
2. Graph Representations
A graph is a collection of nodes and edges:
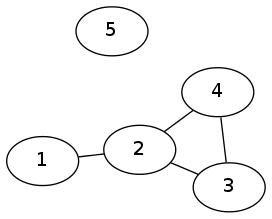
Node | Neighbors |
---|---|
1 | 2 |
2 | 1, 3, 4 |
3 | 2, 4 |
4 | 2, 3 |
5 | (none) |
u_graph = { 1: set([2]),
2: set([1, 3, 4]),
3: set([2, 4]),
4: set([2, 3]),
5: set()}
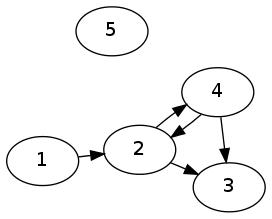
Node | Incident To |
---|---|
1 | 2 |
2 | 3, 4 |
3 | (none) |
4 | 2, 3 |
5 | (none) |
digraph = { 1: set([2]),
2: set([3, 4]),
3: set([]),
4: set([3, 2]),
5: set()}
3. Python Style
3.1 Doc strings
Every function you write for 182 (and for your future class and for the rest of your life!) should be """documented"""!
def add(number1, number2):
"""Returns the sum of number1 and number2."""
return number1 + number2
3.2 Testing
Every function you write for 182 (and for...) should be tested!
def add(number1, number2):
"""Returns the sum of number1 and number2."""
return number1 + number2
def test_add():
"""Tests the add function."""
...
Leave your test code in your solutions. Do not delete it. You may comment it out, if you feel it necessary, but we will look for evidence of testing.
3.3 Commenting
Doc strings tell what the functions are doing. Comments
tell how it is being done. Do not comment simple code (the
above add
function needs no comments). Do
comment complex algorithms and code structures.