Class kochModel.factory.MakeManyAlgo
- class MakeManyAlgo
- extends Object
- implements lrs.IAlgo
This IAlgo constructs a list of Koch curves when it executed on a list of Point2D.Double prototype points.
It does so by Affine transforming (rotating, scaling and translating) the prototype points into the specified line segment.
This algorithm uses an AffineData object that carries the previous prototype point that was processed, plus an AffineXForm object
that was constructed using the Koch curve line segment being replaced. When the algorithm is first executed on the prototype
list, it is assumed that the AffineData object carries a previous point that is (0.0, 0.0).

endPoint

makeKoch
(AffineData, Double)
- A "helper" function for nullCase() and nonNullCase() that will take the AffineData object and the present point and returns a new base case Koch object
nonNullCase
(LRStruct, Object)
- This method is called whenever a specified point of the prototype list is being processed (i
nullCase
(LRStruct, Object)
- This method is called when the end of the list of prototype points is encountered
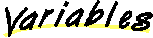
endPoint
Double endPoint = new Point2D.Double(1.0,0.0)
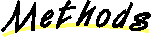
nullCase
public Object nullCase(LRStruct host, Object param)
- This method is called when the end of the list of prototype points is encountered.
The input parameter, param, is assumed to be a AffineData object, which stores the previous point processed and
an AffineXForm object. The enpoint property (1.0, 0,0) is used as the last prototype point.
The AffineXForm object is used to transform the prototype interval into the curve's interval.
A LRStruct with one Koch curve in it is generated, where the Koch curve is over the interval from the previous point to the endpoint.
*
- Parameters:
- host - The LRStruct that is the current node.
- param - The AffineData object carrying the previous point and the AffineXForm object.
- Returns:
- A LRSTruct with one Koch curve in it.
nonNullCase
public Object nonNullCase(LRStruct host, Object param)
- This method is called whenever a specified point of the prototype list is being processed (i.e. the list is not empty yet).
A new base case Koch object is created using the Affine translated points from the prototype and inserted as first into the list
returned by the recursive call to the rest of the prototype list, having replaced the previous point in the AffineData object with the present point..
- Parameters:
- host - The LRStruct that is the current node.
- param - An AffineData object carrying important information.
- Returns:
- A LRStruct filled with Koch curves.
makeKoch
private Koch makeKoch(AffineData ad, Double x)
- A "helper" function for nullCase() and nonNullCase() that will take the AffineData object and the present point and returns a new base case Koch object.
- Parameters:
- ad - The AffineData object needed.
- x - The present point being processed.
- Returns:
- A new Koch object.