Class brsVisitor.BRSDisplayAdapter
- public class BRSDisplayAdapter
- extends Object
This class acts as an MVC view adapter for a BiTree to display the tree on a JComponent. The JComponent is assumed to have a null layout.
The display process clears the JComponent of all other components and then lays out JLabels on it where each label's text is the String representation
of a node's data. Note: If a JScrollPane is used to hold the JComponent, it must be validated after running showTree() method.

colOffset
- The offset from the left in pixels of the entire display
container
- The JComponent upon which the BiTree is to be shown
displayVisitor
- An anonymous inner class IBRSAlgo that is used to traverse the BiTree, calling the showData() method at every non-null node
getMaxDepthVisitor
labelHeight
- The height of the label to make
labelWidth
- The width of the label to make
maxDepth
- The maximum depth, in rows, of the tree to be displayed
rowOffset
- The offset from the top in pixels of the entire display

BRSDisplayAdapter
(JComponent, int, int)
- Constructor for the class that defaults the label width x height to 100 x 30
BRSDisplayAdapter
(JComponent, int, int, int, int)
- Constructor for the class that also allows the label width and height to be specified

displayTree
(BiTree)
- Displays a BiTree on a JComponent by clearing the JComponent of all other components and then laying out a JLabel for every non-null node of the tree
getBounds
(RowColIdx)
- Calculates the bounds of the label for a node, given its row and column index
showData
(Object, RowColIdx)
- Creates a JLabel whose text is the String representation of the supplied data
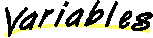
rowOffset
private int rowOffset = 0
- The offset from the top in pixels of the entire display.
colOffset
private int colOffset = 0
- The offset from the left in pixels of the entire display.
labelWidth
private int labelWidth = 100
- The width of the label to make.
labelHeight
private int labelHeight = 30
- The height of the label to make.
maxDepth
private int maxDepth = 0
- The maximum depth, in rows, of the tree to be displayed. This quantitiy is calculated each time the tree is displayed.
container
private JComponent container
- The JComponent upon which the BiTree is to be shown.
displayVisitor
private IVisitor displayVisitor = new IVisitor() {
public Object emptyCase(BiTree host, Object param)
{
return (null);
}
public final Object nonEmptyCase(BiTree host, Object param)
{
showData( host.getRootDat(), (RowColIdx) param);
host.getLeftSubTree().execute(this, ((RowColIdx) param).nextLeft());
host.getRightSubTree().execute(this,((RowColIdx) param).nextRight());
return(null);
}
}
- An anonymous inner class IBRSAlgo that is used to traverse the BiTree, calling the showData() method at every non-null node.
getMaxDepthVisitor
private BRSGetMaxDepthVisitor getMaxDepthVisitor = new BRSGetMaxDepthVisitor()

BRSDisplayAdapter
private BRSDisplayAdapter(JComponent container, int rowOffset, int colOffset)
- Constructor for the class that defaults the label width x height to 100 x 30
- Parameters:
- container - The JComponent to display the BiTree on.
- rowOffset - The vertical offset of the display in pixels.
- colOffset - The horizontal offset of the display in pixels.
BRSDisplayAdapter
public BRSDisplayAdapter(JComponent container, int rowOffset, int colOffset, int labelWidth, int labelHeight)
- Constructor for the class that also allows the label width and height to be specified..
- Parameters:
- container - The JComponent to display the BiTree on.
- rowOffset - The vertical offset of the display in pixels.
- colOffset - The horizontal offset of the display in pixels.
- labelWidth - The width of the labels
- labelHeight - The hieght of the labels.
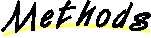
getBounds
private Rectangle getBounds(RowColIdx rowColIdx)
- Calculates the bounds of the label for a node, given its row and column index.
- Parameters:
- rowColIdx - The row and column index of the node.
- Returns:
- A bounds Rectangle object.
showData
private void showData(Object data, RowColIdx rowColIdx)
- Creates a JLabel whose text is the String representation of the supplied data. The bounds of
the label are calculated from the supplied RowColIdx.
- Parameters:
- data - The data to be displayed.
- rowColIdx - The row and column index of the data's node.
displayTree
public void displayTree(BiTree tree)
- Displays a BiTree on a JComponent by clearing the JComponent of all other components and then laying out a JLabel for every non-null node of the tree.
- Parameters:
- tree -