Class lrs.ANode
- abstract class ANode
- extends Object
Represents the abstract list state. Has a concrete toString () method that
uses anynomous inner classes to compute the String representation of the
LRStruct owner.
- Author:
- Dung X. Nguyen Copyright 2001 - All rights reserved.

execute
(IAlgo, Object, LRStruct)
- Executes a visitor algorithm and returns the output
getFirst
(LRStruct)
- Returns the first data object of the referencing LRStruct
getRest
(LRStruct)
- Returns the tail LRStruct of the referencing LRStruct
insertFront
(Object, LRStruct)
- Inserts a data object at the front of the LRStruct owner
removeFront
(LRStruct)
- Removes and returns the first data object for the referencing LRStruct
setFirst
(Object, LRStruct)
- Sets a new first data object for the referencing LRStruct
setRest
(LRStruct, LRStruct)
- Sets a new tail for the referencing LRStruct
toString
(LRStruct)
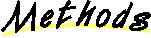
toString
String toString(LRStruct owner)
getRest
abstract LRStruct getRest(LRStruct owner)
- Returns the tail LRStruct of the referencing LRStruct.
- Parameters:
- owner - the LRStruct referencing this ANode.
- Returns:
- the tail LRStruct of owner.
getFirst
abstract Object getFirst(LRStruct owner)
- Returns the first data object of the referencing LRStruct.
- Parameters:
- owner - the LRStruct referencing this ANode.
- Returns:
- the tail LRStruct of owner.
setRest
abstract LRStruct setRest(LRStruct tail, LRStruct owner)
- Sets a new tail for the referencing LRStruct.
- Parameters:
- tail - the new tail for the owner LRStruct.
- owner - the LRS referencing this ANode.
- Returns:
LRStruct
owner
setFirst
abstract LRStruct setFirst(Object dat, LRStruct owner)
- Sets a new first data object for the referencing LRStruct.
- Parameters:
- first - the new data object for this ANode.
- owner - the LRS referencing this ANode.
- Returns:
LRStruct
owner
insertFront
abstract LRStruct insertFront(Object dat, LRStruct owner)
- Inserts a data object at the front of the LRStruct owner.
- Parameters:
- dat - the object to be inserted at the front.
- owner - the LRS referencing this ANode.
- Returns:
LRStruct
owner
removeFront
abstract Object removeFront(LRStruct owner)
- Removes and returns the first data object for the referencing LRStruct.
- Parameters:
- owner - the LRS referencing this ANode.
- Returns:
- the front data of the LRStruct owner.
execute
abstract Object execute(IAlgo algo, Object inp, LRStruct owner)
- Executes a visitor algorithm and returns the output.
- Parameters:
- algo - the visitor algorithm to be executed.
- inp - the input needed by the algorithm.
- owner - the LRStruct referencing this ANode.