Class lrs.EmptyNode
- class EmptyNode
- extends lrs.ANode
Represents the empty state of a mutable list LRStruct. Uses the
Singleton pattern.
- Author:
- Dung X. Nguyen Copyright 2001 - All rights reserved.

Singleton
- Singleton Pattern

EmptyNode
()

execute
(IAlgo, Object, LRStruct)
- Calls the
IAlgo
visitor's empty case
getFirst
(LRStruct)
- Throws java
getRest
(LRStruct)
- Throws java
insertFront
(Object, LRStruct)
- The owner becomes non-empty and has dat as its first element
removeFront
(LRStruct)
- Throws java
setFirst
(Object, LRStruct)
- Throws java
setRest
(LRStruct, LRStruct)
- Throws java
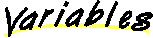
Singleton
static final EmptyNode Singleton = new EmptyNode()
- Singleton Pattern.

EmptyNode
private EmptyNode()
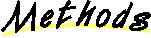
getRest
LRStruct getRest(LRStruct owner)
- Throws java.util.NoSuchElementException.
getFirst
Object getFirst(LRStruct owner)
- Throws java.util.NoSuchElementException.
setRest
LRStruct setRest(LRStruct tail, LRStruct owner)
- Throws java.util.NoSuchElementException.
setFirst
LRStruct setFirst(Object dat, LRStruct owner)
- Throws java.util.NoSuchElementException.
insertFront
LRStruct insertFront(Object dat, LRStruct owner)
- The owner becomes non-empty and has dat as its first element.
removeFront
Object removeFront(LRStruct owner)
- Throws java.util.NoSuchElementException.
execute
Object execute(IAlgo algo, Object inp, LRStruct owner)
- Calls the
IAlgo
visitor's empty case.