Class lrs.LRStruct
- public class LRStruct
- extends Object
Mutable linear recursive structure.
Visitor Pattern: Serves as a host capable of executing algorithms which are
visitors.
- Author:
- Dung X. Nguyen Copyright 2001 - All rights reserved.

_head
- The state of of this
LRStruct

LRStruct
()
- Initializes this
LRStruct
to the empty state
LRStruct
(ANode)
- Initiazes this
LRStruct
with a given head node

execute
(IAlgo, Object)
- Hook method to execute an algorithm with a given input and return
getFirst
()
- Gets the first data element from this
LRStruct
getHead
()
- Gets the head node (i
getRest
()
- Gets the rest of this
LRStruct
insertFront
(Object)
- Inserts dat to the front of this LRStruct
removeFront
()
- Removes and returns this
LRStruct
's first
setFirst
(Object)
- Sets first data element to a new value
setHead
(ANode)
- Changes the head node (i
setRest
(LRStruct)
- Sets a new tail for this
LRStruct
toString
()
- Returns "()" if empty, otherwise returns the contents of this
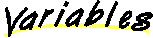
_head
private ANode _head
- The state of of this
LRStruct
.

LRStruct
public LRStruct()
- Initializes this
LRStruct
to the empty state.
LRStruct
LRStruct(ANode node)
- Initiazes this
LRStruct
with a given head node.
- Parameters:
- node - != null.
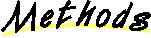
toString
public String toString()
- Returns "()" if empty, otherwise returns the contents of this
LRStruct
enclosed in parentheses.
insertFront
public final LRStruct insertFront(Object dat)
- Inserts dat to the front of this LRStruct.
post condition: getFirst()
now returns dat.
- Parameters:
- dat - data to be inserted.
- Returns:
- this
LRStruct
removeFront
public final Object removeFront()
- Removes and returns this
LRStruct
's first.
getFirst
public final Object getFirst()
- Gets the first data element from this
LRStruct
setFirst
public final LRStruct setFirst(Object dat)
- Sets first data element to a new value.
- Parameters:
- dat - replaces the existing first for this
LRStruct
. - Returns:
- this
LRStruct
getRest
public final LRStruct getRest()
- Gets the rest of this
LRStruct
.
setRest
public final LRStruct setRest(LRStruct tail)
- Sets a new tail for this
LRStruct
.
post condition: getRest()
now returns tail.
- Returns:
- this
LRStruct
execute
public final Object execute(IAlgo algo, Object inp)
- Hook method to execute an algorithm with a given input and return
an appropriate output object.
- Parameters:
- algo - an algorithm (!= null) that operates on this LRStruct.
- inp - input object needed by visitor algo.
- Returns:
- output object resulting from the execution of algo.
setHead
final LRStruct setHead(ANode head)
- Changes the head node (i.e. state) of this
LRStruct
.
- Parameters:
- head - replaces the exisiting state of this
LRStruct
. - Returns:
- this
LRStruct
getHead
final ANode getHead()
- Gets the head node (i.e. state) of this
LRStruct
.
- Returns:
- the head node.