Tutorial 09: AWT Applet
Introduction
This tutorial covers:
- AWT applets
- Combining applets and GUI applications.
Applets are Java programs that are uploaded from the Web server and run on the client
machine inside of a Java-enabled Web browser. We shall restrict ourselves to working
only with Java's AWT (abstract window toolkit) components instead of Swing because most
current browsers do not fully support Swing applets. Just about all the Swing
components would have their own AWT counterparts. For example the AWT counterpart
for JButton is Button. Refer to the UML diagram for Java GUI component in lab #04 to
see where AWT components belong in the taxonomy tree.
I. Sample Program
Create a local directory comp212/tutorials/09
for this lab and copy the
files ~/comp212/tutorials/09/*.java
and ~/comp212/tutorials/09
/AppletOnly.html. These are the Java source code and html document for
an applet that manipulates LRStruct. Compile the Java source and then browse
AppletOnly.html to see the applet run. Play around with this applet to see how it
behaves. The program is designed according to the MVC pattern as shown in the UML
diagram below.
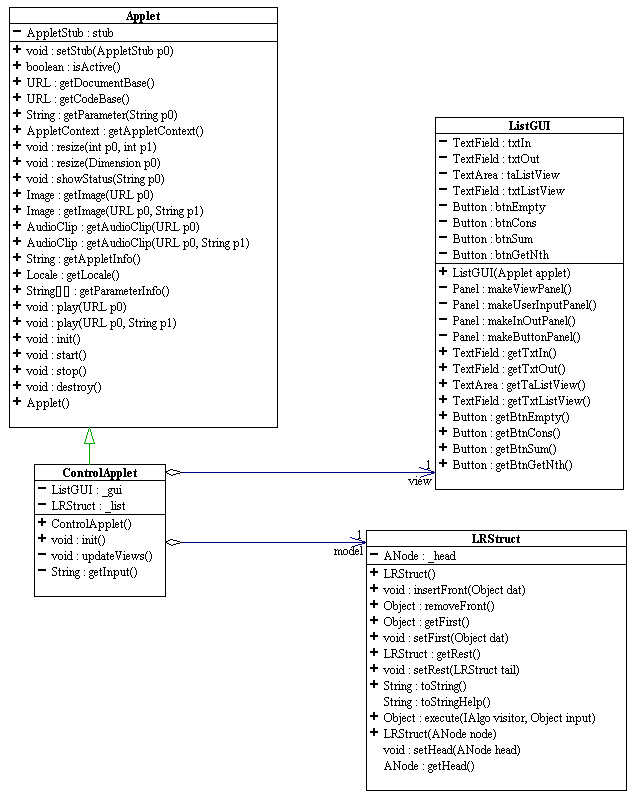
In the above diagram, the model is the familiar LRStruct. ListGUI is the
view. The controller, ControlApplet, is a subclass of java.awt.Applet. It
overrides the init () method to instantiate the view and the model, and add action
listeners to the view's GUI components in order to interact with external users and update
the view accordingly.
II. Basic Applet MVC Design
Model
- The model should be completely independent from the controller and the view. In
the above example, the model is LRStruct with a few of its visitors.
View
- Create a view class with the desired GUI components and appropriate accessor methods for
these components. In the above example, ListGUI is the view with various buttons and
other AWT components.
- Add a constructor with an Applet parameter. In this constructor, add the GUI
components to the Applet parameter. The Applet parameter is in effect the container
for all the GUI components of the view. As an example, here is the code for the
constructor of ListGUI.
public ListGUI (Applet applet)
{
applet.setLayout (new BorderLayout (10, 10));
applet.add (makeViewPanel(), BorderLayout.CENTER);
applet.add (makeUserInputPanel(), "West");
}
Controller
- Subclass Applet - As shown in the above UML diagram, ControlApplet is a subclass of
Applet.
- Add a constructor to instantiate the views and their models. The applet should
pass itself to the constructor of the views as the container for all the GUI components in
the views. In our example, the constructor ControlApplet () calls the Applet default
constructor and then instantiates the GUI view passing itself as the argument.
public ControlApplet ()
{
super (); // calls the Applet default constructor.
_gui = new ListGUI (this); // this Applet
is the container for ListGUI's components.
}
- Override init () if necessary- This should contain code to initialize the applet to a
well-defined state. When a browser opens an html document containing an applet, it
calls the applet init() method. You almost always want to override init().
ControlApplet overrides init() to add action listeners to the view's GUI components.
- Override start() if necessary - The browser will call the applet's start() method after
calling init() to run the applet on the client machine. ControlApplet need not override
start().
- Override stop() if necessary - When the browser no longer displays the page with the
applet, it calls the applet's stop() method. ControlApplet need not override stop().
- Override destroy () if necessary - The browser calls destroy () to free up any resources
that are being used, after calling stop(). ControlApplet need not override
destroy().
- Create and html document with an applet tag to embed the applet in the page. Here
is a simple example of an applet tag: <applet code =
"MyApplet.class" width = 300 height = 200> </applet>.
Use your favorite editor to view AppletOnly.html.
You view an applet by using a Java-enabled browser to open an html document that
contains the appropriate applet tag. You can also use a Java utility that comes with
the JDK called appletViewer to view and test an Applet by executing the command:
appletViewer <html-file-name>.
III. Applet/Application Combination
It is often desirable to have a Java program that can run both as an Applet and as a
stand-alone application. There are many ways to achieve this goal. The
following is a possible approach.
View
- Add a constructor with a Frame parameter that is final.
In this constructor, add the GUI components to the Frame parameter. The Frame
parameter is in effect the container for all the GUI components of the view. Also in
this constructor, add the following anonymous action listener to the Frame parameter.
new WindowAdapter()
{
public void windowClosing(WindowEvent e) {
frame.dispose(); // In order for this inner class
to access frame, frame MUST be final.
System.exit(0);
}
Set the desired Frame size and set the frame's visibility to true.
Controller
- Design the controller as a subclass Applet as in section II. This will allow the
controller to run as an Applet as described in section II.
- Add a constructor to instantiate the views and their models with a Frame parameter.
The Frame parameter should be passed to the constructor of the views as the
container for all the GUI components in the views. Call the initialization
code init() to install the appropriate action listeners.
- Add the main method to instantiate a controller with a Frame instance as an argument to
constructor of the controller. This main method will allow the controller to run as
a stand-alone application.
Exercise: Modify the given ControlApplet.java and ListGUI.java to run
both as an applet and as a stand-alone GUI application by carrying out the steps outlined
in section III.
dxnguyen@cs.rice.edu
revised 03/27/00