Class binaryTree.ANode
- abstract class ANode
- extends Object
Represents the state of the parent binary tree structure. Union pattern
- Author:
- Dung X. Nguyen - Copyright 1999 - All rights reserved.

execute
(IVisitor, Object, BiTree)
- Calls the appropriate visitor's method to execute the visiting algorithm
getLeftSubTree
(BiTree)
- Gets the left subtree of the parent tree
getRightSubTree
(BiTree)
- Gets the right subtree of the parent tree
getRootDat
(BiTree)
- Gets the root data of the parent tree if it exists
insertRoot
(Object, BiTree)
- Inserts a root element to the parent tree
remOurParent
(BiTree, BiTree)
- Removes and return the root element of the grandparent of this node,
remParent
(BiTree, BiTree, BiTree)
- Removes and return the root element of the grandparent of this node,
remRoot
(BiTree)
- Removes and returns the root element from the parent tree
setLeftSubTree
(BiTree, BiTree)
- Sets the left subtree of the parent tree to a given tree
setRightSubTree
(BiTree, BiTree)
- Sets the right subtree of the parent tree to a given tree
setRootDat
(Object, BiTree)
- Sets the root element of the parent tree to a given data object
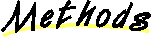
getRootDat
abstract Object getRootDat(BiTree parent)
- Gets the root data of the parent tree if it exists.
- Parameters:
- parent - the context of the state.
- Returns:
- the data element of this node if it exists.
- Throws: NoSuchElementException
- if the parent is empty.
setRootDat
abstract void setRootDat(Object dat, BiTree parent)
- Sets the root element of the parent tree to a given data object.
- Parameters:
- dat -
- parent - the context of this state.
- Throws: NoSuchElementException
- if the parent is empty.
getLeftSubTree
abstract BiTree getLeftSubTree(BiTree parent)
- Gets the left subtree of the parent tree.
- Parameters:
- parent - the context of this state.
- Returns:
- the left subtree of this node if it exists.
- Throws: NoSuchElementException
- if the parent is empty.
getRightSubTree
abstract BiTree getRightSubTree(BiTree parent)
- Gets the right subtree of the parent tree.
- Parameters:
- parent - the context of this state.
- Returns:
- the right subtree of this node if it exists.
- Throws: NoSuchElementException
- if the parent is empty.
setLeftSubTree
abstract void setLeftSubTree(BiTree biTree, BiTree parent)
- Sets the left subtree of the parent tree to a given tree.
Allows for growing the parent tree.
- Parameters:
- biTree - != null.
- parent - the context of this state.
- Throws: NoSuchElementException
- if the parent is empty.
setRightSubTree
abstract void setRightSubTree(BiTree biTree, BiTree parent)
- Sets the right subtree of the parent tree to a given tree. Allows for growing
the parent tree.
- Parameters:
- biTree - != null.
- parent - the context of this state.
- Throws: NoSuchElementException
- if the parent is empty.
insertRoot
abstract void insertRoot(Object dat, BiTree parent)
- Inserts a root element to the parent tree.
Allows for state change from empty to non-empty.
- Parameters:
- dat -
- parent - the context of this state.
- Throws: IllegaStateException
- if the parent is not empty.
remRoot
abstract Object remRoot(BiTree parent)
- Removes and returns the root element from the parent tree.
Allows for state change from non-empty to empty.
- Parameters:
- dat -
- parent - the context of this state.
- Throws: IllegaStateException
- if the parent has more than one element.
remParent
abstract Object remParent(BiTree aunt, BiTree grandPo, BiTree mom)
- Removes and return the root element of the grandparent of this node,
asking the sibbling of the parent tree to do it if necessary.
- Parameters:
- aunt - the sibbling of the parent of this node.
- grandPo - the grand parent of this node.
- mom - the parent (i.e. context) of this node.
- Returns:
- the root data of the grandparent of this node.
remOurParent
abstract Object remOurParent(BiTree grandParent, BiTree parent)
- Removes and return the root element of the grandparent of this node,
knowing that the sibbling of the parent tree is empty.
- Parameters:
- grandParent - the grand parent of this BiTree.
- parent - the context of this node.
- Returns:
- the root data of the grandparent of this node.
execute
abstract Object execute(IVisitor algo, Object input, BiTree parent)
- Calls the appropriate visitor's method to execute the visiting algorithm.
- Parameters:
- algo - the visiting algorithm
- input - the input the algorithm needs.
- parent - the context of this node.
- Returns:
- the output for the algorithm.