Class binaryTree.BiTree
- public class BiTree
- extends Object
Models the binary tree structure using the state pattern and the visitor pattern.
Provides only structural behaviors and a hook to execute any visitor algorithm.
Exercise: Override the toString() method. Use helper methods if necessary.
- Author:
- Dung X. Nguyen - Copyright 1999 - All rights reserved.

_rootNode
- the state of this BiTree

BiTree
()
- Initializes this BiTree to the empty state

execute
(IVisitor, Object)
- Hook to execute any algorithm that presents itself as a visitor to this BiTree
getLeftSubTree
()
- Gets the left subtree of this BiTree if it exists
getRightSubTree
()
- Gets the right subtree of this BiTree if it exsists
getRootDat
()
- Gets the root data of this BiTree if it exists
getRootNode
()
- Returns the current state of this BiTree
insertRoot
(Object)
- Inserts a root element to this BiTree
remOurParent
(BiTree)
- Helper method for
remParent ()
remParent
(BiTree, BiTree)
- Helper method for
remRoot ()
remRoot
()
- Removes and returns the root data element of this BiTree
setLeftSubTree
(BiTree)
- Attaches a new left subtree to the left of this BiTree
setRightSubTree
(BiTree)
- Attaches a new right subtree to the left of this BiTree, allowing this BiTree
setRootDat
(Object)
- Sets the root data element to a given object
setRootNode
(ANode)
- Changes this BiTree to a given new state (i
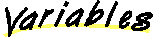
_rootNode
private ANode _rootNode
- the state of this BiTree.

BiTree
public BiTree()
- Initializes this BiTree to the empty state.
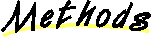
getRootDat
public Object getRootDat()
- Gets the root data of this BiTree if it exists.
- Returns:
- the root data element of this BiTree if it exists.
- Throws: NoSuchElementException
- if this BiTree is empty.
setRootDat
public void setRootDat(Object dat)
- Sets the root data element to a given object.
- Parameters:
- dat -
- Throws: NoSuchElementException
- if this BiTree is empty.
getLeftSubTree
public BiTree getLeftSubTree()
- Gets the left subtree of this BiTree if it exists.
- Returns:
- the left subtree of this BiTree if it exists.
- Throws: NoSuchElementException
- if this BiTree is empty.
getRightSubTree
public BiTree getRightSubTree()
- Gets the right subtree of this BiTree if it exsists.
- Returns:
- the right subtree of this BiTree if it exists.
- Throws: NoSuchElementException
- if this BiTree is empty.
setLeftSubTree
public void setLeftSubTree(BiTree biTree)
- Attaches a new left subtree to the left of this BiTree. Allowing this BiTree
to grow to the left.
- Parameters:
- biTree -
- Throws: NoSuchElementException
- if this BiTree is empty.
setRightSubTree
public void setRightSubTree(BiTree biTree)
- Attaches a new right subtree to the left of this BiTree, allowing this BiTree
to grow to the right.
- Parameters:
- biTree -
- Throws: NoSuchElementException
- if this BiTree is empty.
insertRoot
public void insertRoot(Object dat)
- Inserts a root element to this BiTree.
Allows for state change from empty to non-empty.
- Parameters:
- dat -
- Throws: IllegalStateException
- if this BiTree has more than one element.
remRoot
public Object remRoot()
- Removes and returns the root data element of this BiTree.
- Returns:
- the root data element of this BiTree.
- Throws: NoSuchElementException
- if this BiTree is empty.
execute
public Object execute(IVisitor algo, Object input)
- Hook to execute any algorithm that presents itself as a visitor to this BiTree.
Applies the visitor pattern.
- Parameters:
- algo - a visitor to a BiTree.
- input - the input for the algo visitor.
- Returns:
- the output for the execution of algo.
setRootNode
final void setRootNode(ANode node)
- Changes this BiTree to a given new state (i.e. node).
- Parameters:
- node - a new root node (i.e.state) for this BiTree.
getRootNode
final ANode getRootNode()
- Returns the current state of this BiTree.
- Returns:
- the current root node (state) of this BiTree.
remParent
final Object remParent(BiTree sis, BiTree dad)
- Helper method for
remRoot ()
. Removes and return the root element of
the parent of this Bitree, asking the sibbling tree to do it if necessary.
- Parameters:
- sis - the sibbling of this BiTree.
- dad - the grand parent of this BiTree.
- Returns:
- the root data of the parent of this BiTree.
remOurParent
final Object remOurParent(BiTree dad)
- Helper method for
remParent ()
. Removes and return the root element of
the parent of this Bitree, knowing the sibbling tree is empty.
- Parameters:
- dad - the parent of this BiTree.
- Returns:
- the root data of the parent of this BiTree.