Class binaryTree.NullNode
- class NullNode
- extends binaryTree.ANode
Represents the empty state of a BiTree. Uses the singleton pattern to model
the uniqueness of "emptiness".
- Author:
- Dung X. Nguyen - Copyright 1999 - All rights reserved.

Singleton
- Singleton pattern

NullNode
()

execute
(IVisitor, Object, BiTree)
- Calls the visitor's nullCase () method to execute the visiting algorithm
getLeftSubTree
(BiTree)
- Throws java
getRightSubTree
(BiTree)
- Throws java
getRootDat
(BiTree)
- Throws java
insertRoot
(Object, BiTree)
- Asks the parent tree to set the root node to a new DatNode containing dat,
remOurParent
(BiTree, BiTree)
- Removes and return the root element of the grandparent of this node,
remParent
(BiTree, BiTree, BiTree)
- Removes and return the root element of the grandparent of this node,
remRoot
(BiTree)
- Throws java
setLeftSubTree
(BiTree, BiTree)
- Throws java
setRightSubTree
(BiTree, BiTree)
- Throws java
setRootDat
(Object, BiTree)
- Throws java
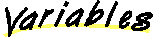
Singleton
public static final NullNode Singleton
- Singleton pattern.

NullNode
private NullNode()
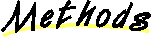
getRootDat
Object getRootDat(BiTree parent)
- Throws java.util.NoSuchElementException.
setRootDat
void setRootDat(Object dat, BiTree parent)
- Throws java.util.NoSuchElementException.
getLeftSubTree
BiTree getLeftSubTree(BiTree parent)
- Throws java.util.NoSuchElementException.
getRightSubTree
BiTree getRightSubTree(BiTree parent)
- Throws java.util.NoSuchElementException.
setLeftSubTree
void setLeftSubTree(BiTree biTree, BiTree parent)
- Throws java.util.NoSuchElementException.
setRightSubTree
void setRightSubTree(BiTree biTree, BiTree parent)
- Throws java.util.NoSuchElementException.
insertRoot
void insertRoot(Object dat, BiTree parent)
- Asks the parent tree to set the root node to a new DatNode containing dat,
resulting in a state change from empty to non-empty.
- Parameters:
- dat -
- parent - the context of this state.
remRoot
Object remRoot(BiTree parent)
- Throws java.util.NoSuchElementException.
remParent
Object remParent(BiTree aunt, BiTree grandPo, BiTree mom)
- Removes and return the root element of the grandparent of this node,
asking the sibbling of the parent tree to do it if necessary.
- Parameters:
- aunt - the sibbling of the parent of this node.
- grandPo - the grand parent of this node.
- mom - the parent (i.e. context) of this node.
- Returns:
- the root data of the grandparent of this node.
remOurParent
Object remOurParent(BiTree grandParent, BiTree parent)
- Removes and return the root element of the grandparent of this node,
knowing the sibbling of the parent tree is empty.
- Parameters:
- grandParent - the grand parent of this BiTree.
- parent - the context of this node.
- Returns:
- the root data of the grandparent of this node.
execute
Object execute(IVisitor algo, Object input, BiTree parent)
- Calls the visitor's nullCase () method to execute the visiting algorithm.
- Parameters:
- algo - the visiting algorithm
- input - the input the algorithm needs.
- parent - the context of this node.
- Returns:
- the output for the nullCase() of the algorithm.