Tutorial 7: Flyweights and Factories
Introduction
This tutorial covers:
- Some details of implementation of the Variable class for programming project 1.
- The flyweight pattern to share objects from a "pool" of objects.
- Factories to manufacture objects instead of calling the objects' constructors directly.
Class Variable
Below is an UML diagram for the design of class Variable.
- Class Variable is both a subclass of ABooleanExp and an implementation of ISNForm. This is the Java specific way of doing
multiple-inheritance. In Java, a class can have only one superclass, but can have as
many super interfaces as desired. Use StructureBuilder to discover the syntax for
mutliple inheritance in Java. As a concrete subclass of ABooleanExp, Variable must have
code for the accept method. As a concrete
"implementation" of ISNForm, Variable must have code for the acceptSNFV
method. Write the code for these methods.
- Class Variable maintains ("has-a") static reference to a QFList,
_symbolTable, and uses it to hold NameVarPair objects which serve no other purpose than pairing a String name with its corresponding Variable
object. The static method Variable makeVariable (String name)
asks _symbolTable to use LookUp,
a singleton visitor to QFList, to find and return a
matching Variable object for a given String name. If the result is null,
then a new NamePair (name, new Variable (name)) is
inserted into _symbolTable and returned. This way,
the next time when the same name is looked up again, the same Variable
(name) object will be returned. The static
method Variable makeVariable
(String name)is called a factory method, and class Variable is said to be a factory that manufactures its own
instances. Since the constructor Variable (String
name) is private, no external clients can instantiate a Variable
object directly. The only way to obtain a Variable
object with a particular String name is to call Variable.makeVariable(name).
Write the code for Variable makeVariable (String name).
- LookUp is an IAlgo
singleton. It knows its QFList host contains NameVarPair objects. It is given as input a String name, and its task is to find and return the matching Variable (name) object in the host QFList.
If there is no match, it returns null (for
non-existence). Write the code for LookUp.
- QFList and IAlgo
constitute the quasi-functional list framework studied in class. QFList serves as a "symbol table" where the pairs
(name, Variable(name)) are stored. Re-use the
framework.
- Class NameVarPair serves no other purpose than pairing a
name with its corresponding Variable object.. As
such, its fields are public and it has no methods. Write the code for NameVarPair.
The Flyweight Pattern
The flyweight pattern is a design pattern used to save space and in some cases time.
To save space, objects of certain type are stored in one place called a storage
"pool". These objects are called flyweights. The flyweight pattern
is used when objects need to be shared because there is expensive to have duplicate copies
and/or because the construction of these objects are expensive. For example, in a
word processing application, there can be hundreds of thousands of "character"
objects. It is thus necessary to define a Character class with a minimal set of
attributes and behaviors and share the Character objects.
A client cannot directly instantiate a flyweight but has to ask a flyweight
"factory" to "vend" it one by calling an appropriate factory method.
A factory class is a class that is capable of manufacturing instances of objects of
certain type. As such, it has access to the constructors of the objects it can
create. When a flyweight factory is asked to vend a particular flyweight, it looks
it up in the storage pool. The flyweight object is returned if found, if not, the
factory instantiates a new flyweight (it has access to the constructor!), adds it to the
pool, and returns it to the calling client. In general the factory maintains a
reference to the storage pool to have quick access to it. In some cases, the factory
serves as the storage pool itself. The high level specification for flyweights and
its factory is "a flyweight factory has (access to) many flyweight objects".
Below is the general UML diagram describing the flyweight pattern.
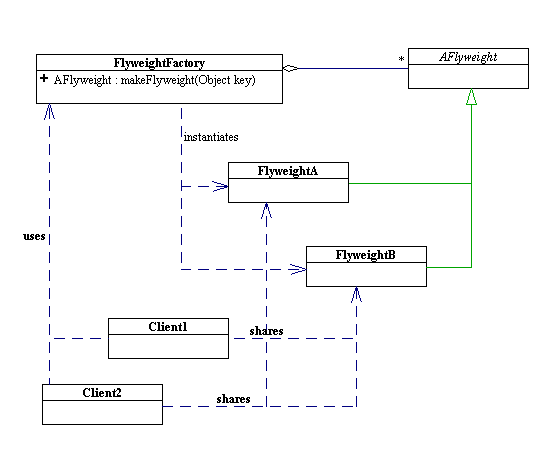
D. X. Nguyen, Oct. 19, 1999.
Copyright 1999, all rights reserved.
dxnguyen@cs.rice.edu