COMP 310
Fall 2012
|
HW01: Simple GUI
Program with Polymorphism
|
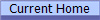 |
In this assignment we will get used to working with the Eclipse environment,
it's various plug-in tools and refresh our skills with polymorphic objects.
This is a warm-up assignment to get you used to the tools and restart your
programming minds and thus, is not indicative of
the skills required for and complexity of future assignments!
You MUST name your homework folders (= Eclipse project folders)
properly. e.g. "HW01", "HW02", etc.! It is strongly
recommended that you create a folder called "Comp310" and place all your
homework folders inside of that folder. For detailed information on
how to set up your homework folders and use Eclipse+Subclipse to turn in
your work, please see Using Eclipse and
Subclipse for Assignment
Turn-In.
Failure to properly name your homework folders WILL result in scoring
deductions on your assignments!
Using Resources
The directions in this assignment (and subsequent assignments) are
deliberately vague in places. The goal is for you to develop your
skills in finding, assembling and utilizing information that is spread across
multiple resources. Here is a
non-exhaustive list of resources to get you started:
- Java Resources
- Comp310 Java Resources
- Oracle/Sun sites
- Eclipse Resources
The Development Process
Software development is an iterative process of
refinement, so we will make our total application piece by piece, sometimes
going back and modifying or perhaps even deleting previous code in order to
accommodate the new features as we add them in.
Never Stray Far From Working Code!
It is a lot easier to find problems in and fix your work
when it is only a small difference from operational code.
- Test often and early! Writing more than a half a dozen lines of
code before checking to make sure everything works is pushing it.
- Fix errors and warnings right away. Don't let them pile up!
- Don't delete old code until the new replacement code is fully
operational. Just comment it out so you can restore it if all
else fails.
Before you start
be sure you have Java, Eclipse and the UML Lab and Subclipse plug-ins
installed!
Make committing to the SVN source control a regular
part of your development process. This has many benefits,
including:
- You can always roll your code back to an earlier, working version.
- You can easily show your work to the staff because the staff has access
to the Comp310 SVN accounts.
- Turning in your work is automatic. Your last submission before the
due date is what will be graded unless prior
arrangements were made.
Before you start, get
Eclipse connected to your Comp310 SVN repository by following the directions on
the Subclipse web page to
- Open a SVN Perspective
- Add a New SVN Repository to View in Subclipse
You are expected to fully
comment all of your code. For more information, see the resources
page on
Commenting in Java.
Make a simple GUI application
In this part of the assignment we will construct a simple
Java GUI application.
Read the following primer
and tutorial FIRST, before starting this part of the assignment!
Never modify the
auto-generated code inside of your initGUI() method unless
explicitly instructed to do so. Even then, limit yourself to only
the indicated changes at the indicated locations! Modifying
code you do not understand is a sure-fire way to break your application!
- Create a new Java Project
- Use the proper naming of your project folder! See above!
- Be sure to make separate source ("src") and class ("bin")
directories.
- Create a package called "view".
- Create a new frame in the view package:
- Right-click the view package and
select New/Other...
- In the dialog box that appears, select
WindowBuilder/Swing Designer/JFrame and click Next
- Type in an appropriately meaningful name for the class.
The "Use advanced template for generate
JFrame" box should be
checked (we will refactor the main()
method to a more appropriate location later).
- Click "Finish".
- Click the green Run button at the top of Eclipse.
- If it asks, select "Java Application"
and the frame class you just made.
- A plain frame with resizing buttons should appear.
- Fix WindowBuilder's bad auto-generated code now!
- (Only necessary if WindowBuilder preferences were not set yet!) Separate the construction of the frame from its initialization:
- Move the auto-generated code out of the constructor into a new
"initGUI()" method.
- Add a call to initGUI() from
inside the constructor.
- Encapsulate the start-up process of the frame and separate it away
from the start-up process of the application:
- Move the call to "setVisible(true)"
from inside of main() to inside of
a new "start()" method.
- Replace the old call to
setVisible(true) with a call to
start().
- Click on the green "Run" button again to make sure your frame still
works.
- This would be a good time to do your first commit to SVN:
- Fix the class "does not declare a static final serialVersionUID field of
type long..." warning.
See the web page
about this.
- Make sure that you can see the graphical representation of your frame in
the WindowBuilder design mode and place two JPanels
onto the frame. Be sure to give the panels meaningful names (i.e. not
the default names!):
- One panel in the "Center" location of the frame (see the
discussion on Layout
Managers).
- Set the panel's background color to something other than the
default gray.
- Run your program. You should see the new panel.
- One panel in the "North" location of the frame.
- It can be difficult to drop the panel so
that it will end up in the frame's North location.
- Set the panel's background color to
contrast with the frame and the other panel.
- Run your program to verify that everything is as you think it
should be.
- Drop a JLabel and a
JButton onto the North panel.
As always, give them meaningful names.
- You can change the names later if you change your mind by using the
"rename" features in Eclipse, which will safely rename the variables and
all references to that variable.
- Run your program. The button won't do anything yet but
change its shade slightly as it is clicked.
- Add an <anonymous> ActionListener to
the button by simply double-clicking the button (same as right-clicking the
button and selecting "add event
handler/action/actionPerformed" or going to the events panel view in
the component's Properties
tab, expanding "action" and double-clicking
"performed".)
- Double-clicking an existing event in a component's
Properties tab will switch to
source-code mode and the cursor
will be placed at the appropriate spot in the code.
- In the empty actionPerformed method
body of the auto-generated ActionListener
for the event, write the line of code that will set the label's text to some new
String.
- Run your code and check if your button does what you want it to do.
For debugging purposes, it might be helpful to have your
actionPerformed code also print some status information to
the console tab whenever the button is clicked.
- Add a JTextField to the
North panel and
modify your button click event code to set the label's text to whatever text
has been typed into the text field. Test your code.
- Override the paintComponent() method of
the center panel to perform some custom painting.
- Click on the center panel on the GUI designer. This
should take you to the line of code where the panel is instantiated.
- Change the instantiation of the JPanel to an anonymous inner class
instance which overrides the paintComponent().
The overridden method will paint a filled colored oval at a fixed
location on the panel:
... = new JPanel() {
/**
* Overridden paintComponent method to paint a shape in the panel.
* @param g The Graphics object to paint on.
**/
public void paintComponent(Graphics g) {
super.paintComponent(g); // Do everything normally done first, e.g. clear the screen.
g.setColor(Color.RED); // Set the color to use when drawing
g.fillOval(75, 100, 20, 40); // paint a filled 20x40 red ellipse whose upper left corner is at (75, 100)
}
};
- You may need to import java.awt.*
to get
the Graphics and
Color class references.
- Fix the class "does not declare a static final serialVersionUID
field of type long..." warning.
See the web page
about this.
Polymorphic Shapes
In this part of the assignment we will construct a
Union
Design Pattern abstract class hierarchy to represent shapes that are capable of
polymorphically drawing themselves onto the screen. We will be
adding this capability to the simple GUI application developed above.
Read the following primer
and tutorial FIRST, before starting this part of the assignment!
Comment the code as you create it!
Fill out the comment boxes when UML Lab presents them to you!
The directions here are deliberately more vague than
above because you should be better versed with the tools plus the emphasis is on
expressing your own ideas about the design and implementation of the shapes.
- Create a shape package in your project.
- Create the abstract share superclass:
- If you have UML Lab installed successfully:
- Create a new, empty UML diagram
- Use UML Lab to create an abstract AShape
superclass
in the shape package:
- Uncheck the box "Constructors from
superclass" to suppress the autogeneration of a constructor for
AShape.
- If you do not have UML Lab, right-click the shape package and
select "New/Class"
- Step 1: Get UML Lab. If all else fails:
- Type in "AShape" as the name of
the new class and check the "abstract"
box. Click "Finish".
- Add any private fields you think that an abstract shape should have.
- Note that below we will write a method to paint the shape at an
arbitrary location -- how does this affect what fields you think the
shape should have?
- Be sure to add comments to the field.
- Add getters and setters for the field as you see fit.
Ask yourself what is reasonable to have and what is not reasonable to
have in your conception of a shape. Be sure to document
this.
- Add the following abstract method: public
abstract void
paint(???) ( the input parameters to be determined by you)
which will paint the shape at a given, specific location.
- Make sure that your documentation clearly spells out what you mean
by "location" with regards to an arbitrary shape.
- You will need to manually add the documentation for the input
parameters to the code.
- Create ONE concrete sub-class of
AShape whose
paint() method draws a specific shape.
- Modify the paintComponent() method of the center panel of your GUI so
that it ALSO paints an AShape at a given location.
- The AShape should be private field of the frame.
- Hard code an instance value for the AShape
field using your concrete sub-class to test that it works at all.
- Do not cover up the original painting output from the previous
section.
- Add a panel on the East, West or South side of the GUI.
- Make it a contrasting color so you can see it.
- Create at least 2 more concrete shape sub-classes.
- Do different shapes need different fields? How does this
affect their constructors?
- Create buttons on the new panel that set the value of the
AShape field to a specific instance of a
shape sub-class
and then call the center panel's repaint() method.
- Clicking on the buttons should change the shape being shown.
- Create a Composite
Design Pattern shape that contains two AShapes and whose
paint
method delegates to each composee.
- Add buttons to demonstrate that you can now draw compound shapes of
arbitrary complexity.
You should have created a UML diagram showing
the entire shape hierarchy (do not include the GUI components).
Grading Critieria (100 pts total)
- Full functionality of all buttons, etc. as described above.
-- 20 pts
- All GUI components are organized as described above -- 10 pts
- UML diagram created -- 5 pts
- Design of abstract shape class (fields and methods) -- 10 pts
- Reasonable class, field, variable and method names. -- 10 pts
- Shapes can be composed appropriately -- 10 pts
- All code you wrote is fully commented -- 20 pts
- Your code produces no warnings -- 10 pts
- Discretionary -- 5 pts
© 2012 by Stephen Wong