COMP 310
Spring 2010
|
HW05:
Self-modifying Finite State Machine
|
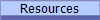 |
The Demo
The demo should load and display automatically.
It is an Honor Code violation to attempt to reverse compile the supplied
demo.
In a nutshell, the demo shows a finite state machine with the ability to
transition from state to state. Run-time selectable options will
modify the state transition behavior. The tool tips in the demo will tell
you most of what everything does.
Descriptons of Selected Operations
- The Make States button will add the designated number
of new states to the system. Every state will always have a unique
identifying index value.
- The Make Transition Algo button will create a
transition algorithm that, when the No-op option is
selected, will create a circular transition path that will cycle through
every available state exactly once.
Note that the transitions may not be in numerical order (to see this, make
about 20 states).
Description of the Options (Be sure to
change back to No-op after you are done inserting or deleting!)
- No-op: The system simply transitions to one of
the available states. The transitions are not modified.
This is considered the default behavior.
- Insert new state: Inserts a new state into the system
and transitions to it. The transitions are modified such that the
current state will always transition by default to the new state and the new
state will transition to where the current state used to transition to.
- Delete state: Removes the next state in the transition
order from the system and changes the transition algo so that the current
state will by default transition to where the deleted state used to
transition to.
The Assignment
In this assignment, you are to replicate the behavior of
this demo. You will deliberately be given less direction in this assignment, as
you will be expected to take on more of the design decisions and implementations
yourself.
You will be using Dr. Wong's extended visitor design pattern implementation,
whose code will be supplied. It should be noted that the generics
specifications of this implementation should be considered a "work in progress"
and any suggestions for improvements are most welcome. As the required
generics specifications are non-trivially complicated and can have subtle
concequences, it is highly recommended that any suggestions be approved by Dr.
Wong before you attempt to use them in your assignment code.
Read the extended visitor documentation
here. (Note: includes the documentation for the supplied
GetIdxAlgo and ILambda
classes -- see below)
UML Diagram of the Extended Visitor Design Pattern
Implementation
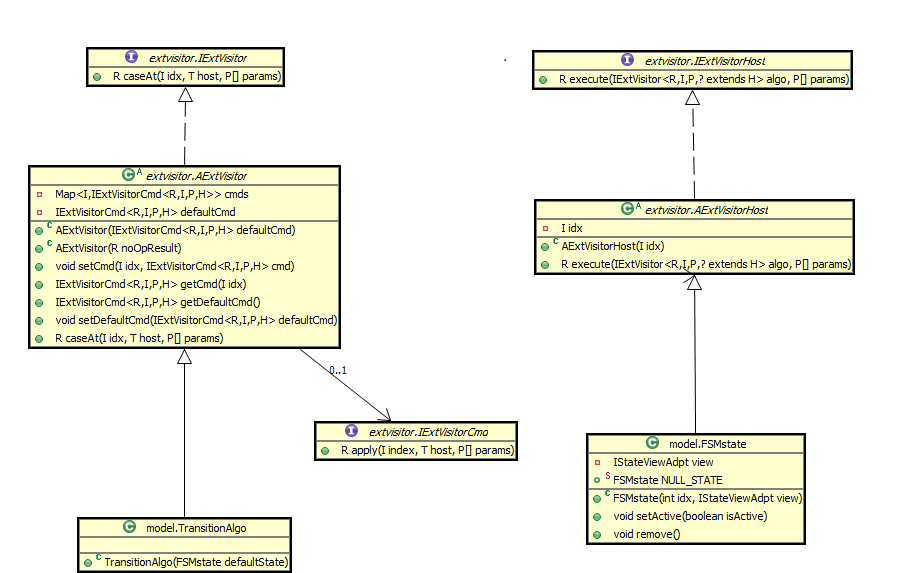
Download the supplied code here.
Assignment Notes:
Model
- In the self-modifying finite state machine, each state is an extended visitor
host, labelled by its identifying index.
- The transitions from one state to another are represented by an extended
visitor that when executed by the current state, returns the next state.
- While it is possible to create an implementation that mutates the active
state into the next state, the management of an informative and intuitive
view can be under that scenario can be somewhat tricky and involved --
please let the staff know if plan to persue that sort of implementation.
- A very convenient manner of storing states such that they can be easily
accessed by their index values is
Map (dictionary) structure, of which the most common
implementation is
HashMap.
- A Map associates a value (the state)
with a key (the index value). Given a key, the associated
value can be retrieved.
- A Map is defined by two types: the
type of its key (K) and the type of its
value (V).
- Maps supply methods to add and remove
key-value pairs as well as to clear the entire dictionary.
- The variable type should be Map but thje
actual object can be a HashMap.
- aMap.values() will return a collection
of all the values (states, here) in the map. This is very useful
when you want to loop over every state in the map.
- aMap.isEmpty() will tell you whether or
not the map is empty.
- aMap.values().iterator().next() will
return the first state in the map, which is not necessarily in any sort
of numerical order.
- Each state should have a notion of being "active" or "inactive" and that
state should be reflected in the UI where the active state is a different
color.
- Transition algo:
- For any undefined states in the transition algo, the default
behavior should be to transition to an available state or a "null" state
if the states map is empty.
- The system should be able to handle available states that never get
transitioned to. To see this in the demo, after a transition
algo has been made, make more states and watch the no-op transitions.
- Transition Options:
- Since the options for the transition algo are instantiated by the
model, who controls what options are available, they need to dynamically
added to the JComboBox in the view.
- The transition algo should never be checking which option is to be
run and doing different things depending on which option is selected.
The option to run should simply be the selected option, independent of
whatever it is.
- Suggestions:
- Use a counter that keeps track of the total number of states created
since the last time the states were cleared. Any new state
will have an index equal to this count. This eliminates two
hosts having the same index.
- Use private methods to help isolate tasks, such as creating a
transition algo, creating a IExtVisitorCmd
objects, making and managing states, etc.
- First write the transition algo without involving the options.
Once that is working, add the option capability in.
View:
- You are welcome to redesign the GUI so long as it contains the same
functionality in an intuitive, user-friendly manner.
- In adherance with the MVC pattern, the states, even though they are
dynamically generated, should have a separate view that is completely
decoupled from anything in the model.
- Be careful not to replicate the accounting of the available states
and their views. For instance, the view should not need to
keep track of how many states are available.
- In the demo, the states are represented in the view as dynamically
created JLabel objects added to a
JPanel.
- The default FlowLayout of the panel
makes it easy to automatically adjust the positions of the states.
- To insure that the states are displayed properly, after adding or
deleting states to the view, always call the panel's
validate() (re-runs the layout manager) and
repaint() methods.
- Labels and other components already paint themselves when their
container repaints, so no overridden paintComponent
methods are needed anywhere.
- The greyed-out buttons are due to calling that button's
setEnabled() method with the appropriate value when certain
operations occur. This prevents inappropriate interactions.
- The JSpinner component uses a
SpinnerNumberModel to create an available set of
integers from 1 to 100. The following line of code was added to
the autogenerated GUI code (nStatesSpin is the
name of the JSpinner variable):
nStatesSpin.setModel(new SpinnerNumberModel(1, 1, 100,
1));
Assignment requirements:
- Use the extended visitor design pattern to represent the states and
transitions.
- Use MVC throughout, including for individual states. The
model and view should be completely decoupled.
- Transition options are dynamically created.
- The method invoked in the model my the "Step Transition Algo" button,
should do nothing more than:
- Deactivate the current state
- Run the transition algorithm on the current state, assigning the
return value to be the new current state.
- Activate the current state.
- The view should have tool tips to aid the user in using the program.
- View should clearly indicate which state is the active (current) state.
- The transition algo options are dynamically added to the view.
The view should have no knowledge of what options are installed.
- The transition algo should not be testing to see which option has been
selected.
© 2010 by Stephen Wong