COMP 310
Spring 2017
|
HW04: Fishworld
|
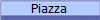 |
Assignment Instructions and Point Values
The assignment is to replicate the
command-dispatching
Fishworld demo.
You must complete the following features:
- The architecture must satisfy HW03 requirements, e.g. must be a Model-View-Controller design with
apprpriately created interfaces and adapters and appropriate methods on each
interface/adapter. (10 pts)
- Painting should be performed as a strategy that the user can select.
(70 pts total)
- The GUI should be updated to enable different painting strategies to
be selected. (10 pts)
- At least 6 different painting strategies should be available, with
at least one choice from each of the 4 types below: (60
pts)
- Non-affine transform-based paint strategy, i.e. directly
implements IPaintStrategy
- Affine transform-based paint strategy:
- Simple geometric shape and/or polygon
- Composite shape that stays upright
- Image
- Implement command-based updating
- The updating of the balls must be done by sending a command whose
purpose is to update the ball (5 pts)
- The ball must be able to accept and execute any command sent to it.
(5 pts)
- You are not required to use the
generic dispatcher but it highly recommended. Get your
HW04 fully operational before switching over
to the generic dispatcher! Use of the generic dispatcher will
be required for the next assignment!
- Full Documentation
- All classes and methods except for those auto-generated from
WindowBuilder.
(5 pts)
- Tooltips on all GUI components ( 5 pts)
Development Process
In this assignment, it is important to be very clear
about exactly what you are doing and the role of
each method you write. On the last assignment, many of the errors
and confusions stemmed for not carefully reading the lecture, lab and resource
materials and following the directions that were outlined there. An
important skill that Comp310 emphasizes is to be able to assemble information
spread across multiple sources and locations into a coherent solution to a
problem. Do not expect that the solution to this assignment can be
found in a single lecture page. Look in the lectures, look in the
labs, look in the resource pages, look at your class lecture notes, look at the
demos and their documentation, even....look
at this page.
A very common mistake to make in this assignment is to
attempt to program the entire painting process. Everything that happens in
an object-oriented program is the combination of invariant and variant pieces.
Remember that the superclasses provide the invariant pieces and the subclasses
provide the variant pieces. In an inheritance hierarchy, as one
moves down the inheritance tree, each subsequent class adds more and more
invariant behavior that its subclasses can use. By the time you are at the
bottom of the inheritance tree, the class is just adding some small piece to a
much larger process, a piece that may in fact be in the middle of that larger
process, so it looks like it isn't accomplishing anything. Most
of the code you will need is already printed on the web pages. Most
subclasses add very little code, perhaps even just supplying parameters to a
superclass constructor.
When writing your own custom paint strategy, first
consider where in the paint strategy hierarchy it should be located.
In general, put it as low on the hierarchy as possible to take advantage of
invariant behavior defined by its superclasses. This minimizes the
amount of code in your class to just its variant part. In fact, your
specific code may reduce to just a very few lines.
So, here are some things to remember:
- IPaintStrategy: The
top-level interface that the Ball uses.
It defines two methods that the Ball must
deal with:
- paint - This
is the replacement for the paint method in the
Ball. Any concrete class that directly implments
IPaintStrategy should have code here
that is essentiatlly exactly what would have gone in the Ball's
paint method.
- init -- This method is used to initialize the paint strategy, if
needed. If no specialized initialization is needed this
method should simply do nothing. The method should be run by
the Ball whenever (make sure you find
all the possibilities!) the Ball
gets a new strategy.
- APaintStrategy: This second-level
abstract class implements an affine transform-based painting process that
uses a Template Method pattern of code for its
paint method. This paint
method is now invariant for all its subclasses, i.e. the subclasses don't
have to implement the paint method anymore,
just the supporting methods for that painting process.
The affine transform-based painting process is essentially what was done in
lab. APaintStrategy
defines an invariant paint method that all its subclasse will inherit and
thus not have to implement. So, in addition to the init method, 2
other methods are defined that sub-classes need to implement if desired:
- paintCfg -
Defined by a subclass if additional processing, e.g. staying upright, is
required before the actual painting takes place in
paintXfrm. This method is part of
the invariant painting process defined by the
paint method. Because subclasses may or may not
do this aditional processing, APaintStrategy
provides default no-op processing for it.
-
paintXfrm - This is
where the actual painting takes place and thus must abstract in
APaintStrategy. Since this
method takes the affine transform as an input parameter, do
not use the internal affine transform to
perform the transformation of the prototype image. Because
this method is independent of the internal affine transform, it contains
only the variant parts of the painting process, ie. the actual painting
of the image using the Graphics object.
- ShapePaintStrategy:
This class build upon and specializes the services provided by
APaintStrategy to better support the
painting of Shape objects. In
particular, the paintXfrm method is
invariant for any Shape.
Subclasses thus do not have to implement
paintXfrm.
-
IShapeFactory:
Shape factories encapsulate and hide the
construction details of the Shape
prototypes used by ShapePaintStrategy.
Input parameters are used to customize the shape.
ShapeFactories can be used in many places
as a quick and easy way to produce Shape
objects.
-
MultiPaintStrategy - This class adds the invariant behaviors specific to a
composite of APaintStrategies.
Besides providing the storage of the references for the strategies in the
composition, it provides the dispatching of
paintXfrm to all the composees (why
paintXfrm and not paint?).
Thus, any subclass of MultiPaintStrategy
need not implement paintXfrm.
-
ImagePaintStrategy:
This APaintStrategy subclass adds
specialized init and
paintXfrm methods to load the image and
calculate a secondary affine transform that is used to help create the the
required additional scaling needed convert the image file into a prototype.
This secondary affine transform is then combined with the supplied affine
transform in paintXfrm to create a net
transform that will enable the image to be painted onto the screen at the
correct location, orientation and size.
It is recommended that you go find your own images to use on the Internet.
GIFs, JPGs, and PNGs will all work. Here are some images to test
with: images.zip.
- Check your scaling calculations using the
FIFA_Soccer_Ball.png image, which has a 0.33 fill factor.
This fill factor should cause the ball to appear to bounce off of its
edge just like a normal ball. The
Earth.png, Jupiter.png,
Mars.png and
Venus.png all have fill factors of 1.0 so they can be used as
secondary checks. (Why are they not useful as primary
checks?)
- Be sure to put your "images"
folder directly below the folder where
ImagePaintStrategy is located as all file location references
will be relative to the folder that contains
ImagePaintStrategy. Thus you can access the files by
writing something like "images\myImage.png"
© 2017 by Stephen Wong