COMP 310
Spring 2017
|
Lec25: Remote
Method Invocation (RMI)
|
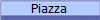 |
Today we will start exploring the world of Remote Method Invocation or "RMI".
The demo code for today can be obtained by creating a
new project in Eclipse and setting the
svn:externals property for the "src"
folder to
"provided https://svn.rice.edu/r/comp310/course/RMIHelloWorld/provided".
The demo code consists of two executable classes, a "client" and a "server"
plus an interface that defines the remote service that the server offers to the
client.
Be sure that the
Server machine has the ServerProcess.REGISTRY_PORT
and ServerProcess.SERVER_PORT open through its
firewall!!
Open ports: 2001-2002 and 2099-2102
Be sure that
launch configuration files for both the client and server have been created!
See the Eclipse Resources web page on
Editing the Launch
Configuration Parameters.
RMI will NOT run unless a proper launch
configuration is created!
To run the demo:
- Highlight the ServerProcess launch
configuration file and click
Run.
- The ServerProcess must be started first,
otherwise the Client will have nothing to
connect to.
- You should see a "Server ready" message
on the console if the Server has started
succesfully.
- Highlight the Client launch
configuration file and click
Run.
- The client should instantiate, connect to the
ServerProcess, print the response from the Server
and exit.
- To connect to a remote machine, edit the launch configuration for
the Client and put the IP address for the
remote server as a "Program Argument".
To stop the running server:
- Change to the server's console by either closing the console of the
client if it open by clicking the "x" on the console tab or by selecting the
server's console by pulling down the "Display Selected Console" drop-list on
the console tab.
- Click the red square "Terminate" button on the console tab.
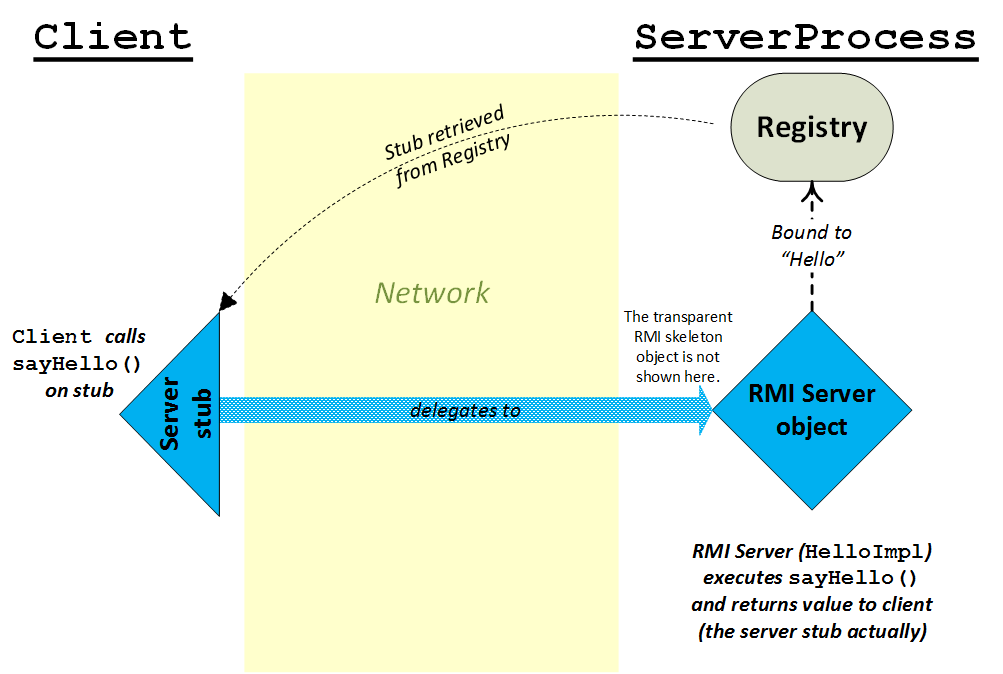
What's going on:
-
The ServerProcess binds a
HelloImpl
stub (an IHello interface implementation) to the name "Hello"
in the Registry.
-
The client asks for the name "Hello"
in the server's (the machine running ServerProcess) Registry and receives the stub to the
HelloImpl object.
-
The client calls sayHello()
on the stub object.
-
The stub transparently delegates the call all the way
across the network to the actual
HelloImpl object
on the server machine.
-
The
HelloImpl object runs
its sayHello() method and returns the result.
-
The stub returns the result across the network back
to the waiting client.
Things to try:
To create your own modifications, simply create
your own Client, ServerProcess,
IHello and HelloImpl classes in your
own package by copying the code from the provided
package and then adjusting the package references.
Do NOT edit the code in the
provided package as you will not be able to commit your code!
- Bind multiple IHello implementations to the
same name in the Registry:
- Start the original ServerProcess.
- Change the return value of sayHello()
method of the HelloImpl class to return a
different value or make a new class implementing
IHello that returns a different value (you will need to modify
ServerProcess to use this new class if you
do this).
- In ServerProcess, change the port value that the
RMI server object (IHello
implementation) is being bound to from
SERVER_PORT to
SERVER_PORT+1 (Otherwise you might get a port conflict error)
- Without terminating the previous
ServerProcess , run the ServerProcess again.
- Without modifying it, run the Client
again.
- Even though the original ServerProcess is
still running, what is the value retrieved by the
Client? Why?
- Bind multiple IHello implementations to
different names in the Registry.
- Start a version of the ServerProcess.
- Run the Client to verify the value
returned is what you expect.
- Modify the IHelloImpl's
sayHello() method to return a different
value or make a new class implementing IHello
that returns a different value (you will need to modify
ServerProcess to use this new class if you
do this).
- In ServerProcess, change the port value that the
RMI server object (IHello
implementation) is being bound to from
SERVER_PORT to
SERVER_PORT+1 (Otherwise you might get a port conflict error)
- Modify the Server's
run() method to bind the
HelloImpl object with a different name.
- Without terminating the previous
ServerProcess , run the ServerProcess again.
- Without modifying it, run the Client
again. What value is returned?
- Modify the Client so that it accesses
the new Registry binding name being used in the modified
ServerProcess code above.
- Run the Client again.
What value is returned?
© 2017 by Stephen Wong